Javascript new Response Tutorial (With Examples)
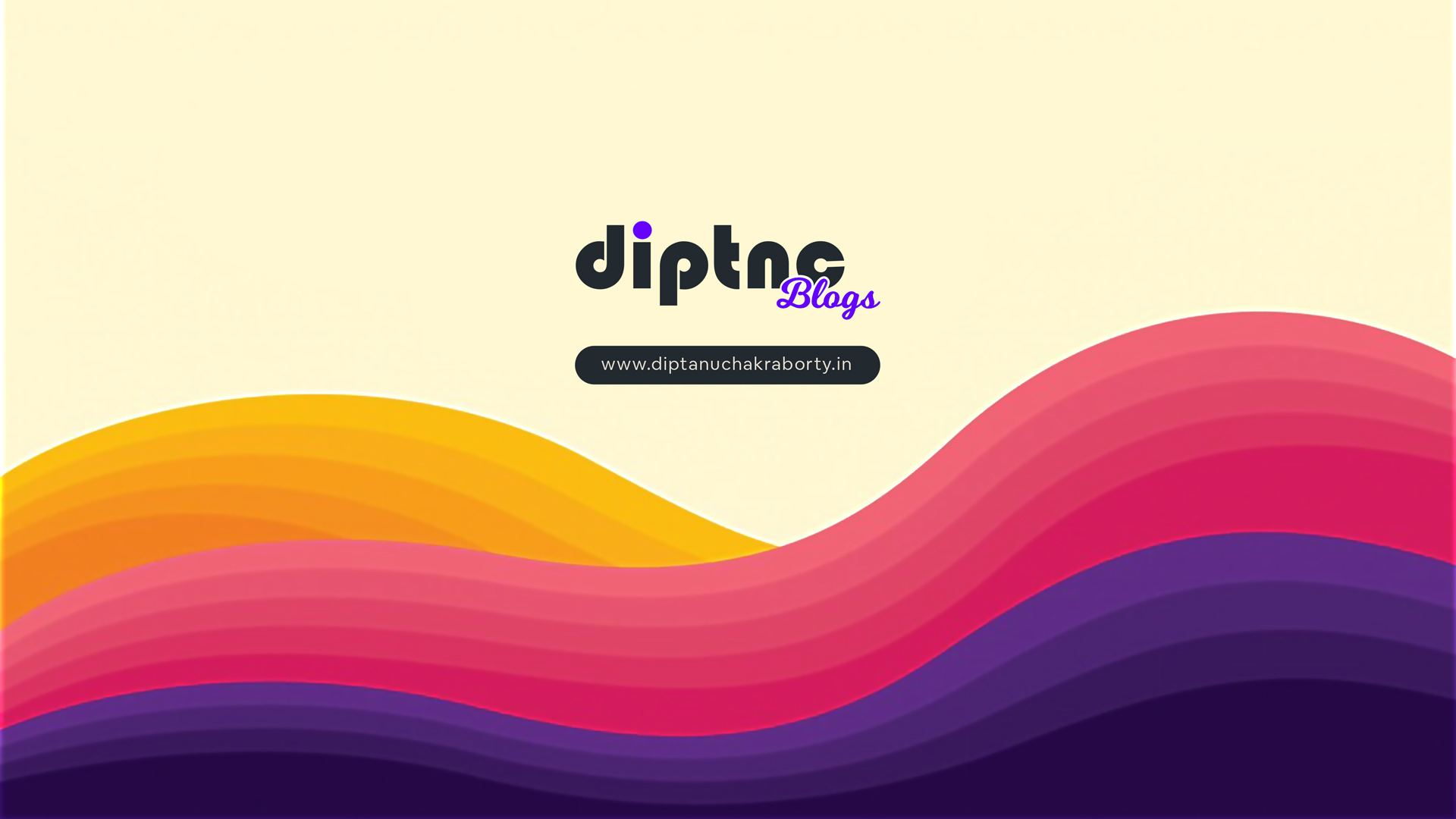
-
Basic Text Response:
You can create a simple text response like this:
javascriptconst text = "Hello, World!"; const response = new Response(text, { status: 200, statusText: "OK", headers: { "Content-Type": "text/plain" } });
-
JSON Response:
To send JSON data as a response:
javascriptconst jsonData = { message: "Success", data: { id: 123, name: "John" } }; const response = new Response(JSON.stringify(jsonData), { status: 200, statusText: "OK", headers: { "Content-Type": "application/json" }, });
-
HTML Response:
If you want to return an HTML response:
javascriptconst html = "<html><body><h1>Welcome to my website</h1></body></html>"; const response = new Response(html, { status: 200, statusText: "OK", headers: { "Content-Type": "text/html" } });
-
Custom Headers:
You can include custom headers in your response:
javascriptconst text = "Custom Headers Example"; const headers = new Headers(); headers.append("Custom-Header", "Some Value"); const response = new Response(text, { status: 200, statusText: "OK", headers });
-
Error Response:
To send an error response with a specific HTTP status code (e.g., 404 Not Found):
javascriptconst errorMessage = "Resource not found"; const response = new Response(errorMessage, { status: 404, statusText: "Not Found", headers: { "Content-Type": "text/plain" } });
These are some basic examples of using the Response constructor to create various types of HTTP responses in JavaScript. You can customize the status code, status text, headers, and response content to suit your needs.